3.2. Numeric Float¶
Represents floating point number (vide IEEE-754)
Could be both signed and unsigned
Default
float
size is 64 bitPython automatically extends
float
when need bigger number
>>> data = 1.337
>>> data = +1.337
>>> data = -1.337
Floating-point numbers are not real numbers, so the result of 1.0/3.0
cannot be represented exactly without infinite precision. In the decimal
(base 10) number system, one-third is a repeating fraction, so it has an
infinite number of digits. Even simple non-repeating decimal numbers can
be a problem. One-tenth (0.1) is obviously non-repeating, so we can express
it exactly with a finite number of digits. As it turns out, since numbers
within computers are stored in binary (base 2) form, even one-tenth cannot
be represented exactly with floating-point numbers,
When should you use integers and when should you use floating-point numbers? A good rule of thumb is this: use integers to count things and use floating-point numbers for quantities obtained from a measuring device. As examples, we can measure length with a ruler or a laser range finder; we can measure volume with a graduated cylinder or a flow meter; we can measure mass with a spring scale or triple-beam balance. In all of these cases, the accuracy of the measured quantity is limited by the accuracy of the measuring device and the competence of the person or system performing the measurement. Environmental factors such as temperature or air density can affect some measurements. In general, the degree of inexactness of such measured quantities is far greater than that of the floating-point values that represent them.
Despite their inexactness, floating-point numbers are used every day throughout the world to solve sophisticated scientific and engineering problems. The limitations of floating-point numbers are unavoidable since values with infinite characteristics cannot be represented in a finite way. Floating-point numbers provide a good trade-off of precision for practicality.
Note
Source [1]
3.2.1. Without Zero Notation¶
.44
- notation without leading zero69.
- notation without trailing zeroUsed by
numpy
Leading zero:
>>> data = .44
>>> print(data)
0.44
Trailing zero:
>>> data = 69.
>>> print(data)
69.0
3.2.2. Engineering Notation¶
The exponential is a number divisible by 3
Allows the numbers to explicitly match their corresponding SI prefixes
The E (or e) should not be confused with the exponential
e
which holds a completely different significance
Name |
Symbol |
Base |
Value |
---|---|---|---|
yotta |
Y |
1e24 |
1000000000000000000000000.0 |
zetta |
Z |
1e21 |
1000000000000000000000.0 |
exa |
E |
1e18 |
1000000000000000000.0 |
peta |
P |
1e15 |
1000000000000000.0 |
tera |
T |
1e12 |
1000000000000.0 |
giga |
G |
1e9 |
1000000000.0 |
mega |
M |
1e6 |
1000000.0 |
kilo |
k |
1e3 |
1000.0 |
1e0 |
1.0 |
||
milli |
m |
1e−3 |
0.001.0 |
micro |
μ |
1e−6 |
0.000001.0 |
nano |
n |
1e−9 |
0.000000001.0 |
pico |
p |
1e−12 |
0.000000000001.0 |
femto |
f |
1e−15 |
0.000000000000001.0 |
atto |
a |
1e−18 |
0.000000000000000001.0 |
zepto |
z |
1e−21 |
0.000000000000000000001.0 |
yocto |
y |
1e−24 |
0.000000000000000000000001.0 |
>>> x = 1e6
>>> print(x)
1000000.0
>>>
>>> x = 1E6
>>> print(x)
1000000.0
>>> x = +1e6
>>> print(x)
1000000.0
>>>
>>> x = -1e6
>>> print(x)
-1000000.0
>>> x = 1e-3
>>> print(x)
0.001
>>>
>>> x = 1e-6
>>> print(x)
1e-06
3.2.3. Scientific notation¶
The E (or e) should not be confused with the exponential
e
which holds a completely different significance
>>> 1e1
10.0
>>>
>>> 1e2
100.0
>>>
>>> 1e3
1000.0
>>> 1e-3
0.001
>>>
>>> 1e-4
0.0001
>>>
>>> 1e-5
1e-05
>>>
>>> 1e-6
1e-06
>>> 1e3
1000.0
>>>
>>> -1e3
-1000.0
>>>
>>> 1e-3
0.001
>>>
>>> -1e-3
-0.001
>>> 1.337 * 1e3
1337.0
>>>
>>> 1.337 * 1e-3
0.001337
>>> 1.337e3
1337.0
>>>
>>> 1.337e-3
0.001337
>>>
>>> 1.337e-4
0.0001337
>>> 1.337e-5
1.337e-05
3.2.4. Type Conversion¶
Builtin function float()
converts argument to float
>>> float(1)
1.0
>>>
>>> float(+1)
1.0
>>>
>>> float(-1)
-1.0
>>> float(1.337)
1.337
>>>
>>> float(+1.337)
1.337
>>>
>>> float(-1.337)
-1.337
>>> float('1.337')
1.337
>>>
>>> float('+1.337')
1.337
>>>
>>> float('-1.337')
-1.337
>>> float('1,337')
Traceback (most recent call last):
ValueError: could not convert string to float: '1,337'
>>> float('+1,337')
Traceback (most recent call last):
ValueError: could not convert string to float: '+1,337'
>>> float('-1,337')
Traceback (most recent call last):
ValueError: could not convert string to float: '-1,337'
3.2.5. Round Number¶
Rounding a number
>>> pi = 3.14159265359
>>>
>>>
>>> round(pi, 4)
3.1416
>>>
>>> round(pi, 2)
3.14
>>>
>>> round(pi)
3
>>>
>>> round(pi, 0)
3.0
Rounding a number in string formatting
>>> pi = 3.14159265359
>>>
>>>
>>> print(f'Pi number is {pi}')
Pi number is 3.14159265359
>>>
>>> print(f'Pi number is {pi:f}')
Pi number is 3.141593
>>>
>>> print(f'Pi number is {pi:.4f}')
Pi number is 3.1416
>>>
>>> print(f'Pi number is {pi:.2f}')
Pi number is 3.14
>>>
>>> print(f'Pi number is {pi:.0f}')
Pi number is 3
>>> round(10.5)
10
>>>
>>> round(10.51)
11
3.2.6. Type Checking¶
>>> x = 1.2
>>>
>>> type(x)
<class 'float'>
>>>
>>> x = 1.2
>>> type(x) is float
True
>>>
>>> type(x) in (int, float)
True
>>> x = 1.2
>>>
>>> isinstance(x, float)
True
>>>
>>> isinstance(x, (int,float))
True
>>>
>>> isinstance(x, int|float) # since 3.10
True
3.2.7. References¶
3.2.8. Assignments¶
"""
* Assignment: Type Float Tax
* Type: class assignment
* Complexity: easy
* Lines of code: 5 lines
* Time: 3 min
English:
1. Cost of the service is 100.00 PLN net
2. Service has value added tax (VAT) rate of 23%
3. Calculate tax and gross values
4. To calculate tax, multiply net times VAT
5. To calculate gross multiply net times VAT plus 1
6. Result must be in PLN
7. Mind the operator precedence
8. Run doctests - all must succeed
Polish:
1. Cena usługi wynosi 100.00 PLN netto
2. Usługa objęta jest 23% stawką VAT
3. Oblicz wartości podatku oraz cenę brutto
4. Aby obliczyć podatek, pomnóż cenę netto razy stawkę VAT
5. Aby obliczyć cenę brutto pomnóż cenę netto razy stawka VAT plus 1
6. Wynik musi być w PLN
7. Zwróć uwagę na kolejność wykonywania działań
8. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert net is not Ellipsis, \
'Assign your result to variable `net`'
>>> assert tax is not Ellipsis, \
'Assign your result to variable `tax`'
>>> assert gross is not Ellipsis, \
'Assign your result to variable `gross`'
>>> assert type(net) is float, \
'Variable `net` has invalid type, should be float'
>>> assert type(tax) is float, \
'Variable `tax` has invalid type, should be float'
>>> assert type(gross) is float, \
'Variable `gross` has invalid type, should be float'
>>> net / PLN
100.0
>>> tax / PLN
23.0
>>> gross / PLN
123.0
"""
PLN = 1.00
VAT_23 = 23 / 100
# 100.0 PLN, without tax
# type: float
net = ...
# 23% of net
# type: float
tax = ...
# Gross is net plus tax in PLN
# type: float
gross = ...
"""
* Assignment: Type Float Altitude
* Type: class assignment
* Complexity: easy
* Lines of code: 3 lines
* Time: 3 min
English:
1. Plane altitude is 10.000 ft
2. Data uses imperial (US) system
3. Convert to metric (SI) system
4. Result round to one decimal place
5. Run doctests - all must succeed
Polish:
1. Wysokość lotu samolotem wynosi 10 000 ft
2. Dane używają systemu imperialnego (US)
3. Przelicz je na system metryczny (układ SI)
4. Wynik zaokrąglij do jednego miejsca po przecinku
5. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert altitude is not Ellipsis, \
'Assign your result to variable `altitude`'
>>> assert altitude_m is not Ellipsis, \
'Assign your result to variable `altitude_m`'
>>> assert altitude_ft is not Ellipsis, \
'Assign your result to variable `altitude_ft`'
>>> assert type(altitude) is float, \
'Variable `altitude` has invalid type, should be float'
>>> assert type(altitude_m) is float, \
'Variable `altitude_m` has invalid type, should be float'
>>> assert type(altitude_ft) is float, \
'Variable `altitude_ft` has invalid type, should be float'
>>> altitude
3048.0
>>> altitude_m
3048.0
>>> altitude_ft
10000.0
"""
m = 1
ft = 0.3048 * m
# 10_000 ft
# type: float
altitude = ...
# Altitude in meters
# type: float
altitude_m = ...
# Altitude in feet
# type: float
altitude_ft = ...
"""
* Assignment: Type Float Volume
* Type: class assignment
* Complexity: easy
* Lines of code: 4 lines
* Time: 3 min
English:
1. Bottle volume is 20 Fl Oz
2. Data uses imperial (US) system
3. Convert to metric (SI) system
4. Run doctests - all must succeed
Polish:
1. Objętość butelki wynosi 20 Fl Oz
2. Dane używają systemu imperialnego (US)
3. Przelicz je na system metryczny (układ SI)
4. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert volume is not Ellipsis, \
'Assign your result to variable `volume`'
>>> assert volume_floz is not Ellipsis, \
'Assign your result to variable `volume_floz`'
>>> assert volume_l is not Ellipsis, \
'Assign your result to variable `volume_l`'
>>> assert type(volume) is float, \
'Variable `volume` has invalid type, should be float'
>>> assert type(volume_floz) is float, \
'Variable `volume_floz` has invalid type, should be float'
>>> assert type(volume_l) is float, \
'Variable `volume_l` has invalid type, should be float'
>>> volume_floz
20.0
>>> volume_l
0.5914688
"""
liter = 1
floz = 0.02957344 * liter
# 20 Fl Oz
# type: float
volume = ...
# Volume in fluid ounces
# type: float
volume_floz = ...
# Volume in liters
# type: float
volume_l = ...
"""
* Assignment: Type Float Euler
* Type: class assignment
* Complexity: easy
* Lines of code: 4 lines
* Time: 5 min
English:
1. Euler's number is 2.71828
2. Round number using f-string formatting
3. Run doctests - all must succeed
Polish:
1. Liczba Eulra to 2.71828
2. Zaokrąglij liczbę wykorzystując formatowanie f-string
3. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert a is not Ellipsis, \
'Assign your result to variable `a`'
>>> assert b is not Ellipsis, \
'Assign your result to variable `b`'
>>> assert c is not Ellipsis, \
'Assign your result to variable `c`'
>>> assert d is not Ellipsis, \
'Assign your result to variable `d`'
>>> assert type(a) is str, \
'Variable `a` has invalid type, should be str'
>>> assert type(b) is str, \
'Variable `b` has invalid type, should be str'
>>> assert type(c) is str, \
'Variable `c` has invalid type, should be str'
>>> assert type(d) is str, \
'Variable `d` has invalid type, should be str'
>>> a
"Euler's number with 0 decimal places: 3"
>>> b
"Euler's number with 1 decimal places: 2.7"
>>> c
"Euler's number with 2 decimal places: 2.72"
>>> d
"Euler's number with 3 decimal places: 2.718"
"""
EULER = 2.71828
# Euler's number with 0 decimal places
# type: str
a = f"Euler's number with 0 decimal places: {EULER}"
# Euler's number with 1 decimal places
# type: str
b = f"Euler's number with 1 decimal places: {EULER}"
# Euler's number with 2 decimal places
# type: str
c = f"Euler's number with 2 decimal places: {EULER}"
# Euler's number with 3 decimal places
# type: str
d = f"Euler's number with 3 decimal places: {EULER}"
"""
* Assignment: Type Float Velocity
* Type: class assignment
* Complexity: easy
* Lines of code: 9 lines
* Time: 3 min
English:
1. Speed limit is 75 MPH
2. Data uses imperial (US) system
3. Convert to metric (SI) system
4. Speed limit print in KPH (km/h)
5. Run doctests - all must succeed
Polish:
1. Ograniczenie prędkości wynosi 75 MPH
2. Dane używają systemu imperialnego (US)
3. Przelicz je na system metryczny (układ SI)
4. Ograniczenie prędkości wypisz w KPH (km/h)
5. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert kph is not Ellipsis, \
'Assign your result to variable `kph`'
>>> assert mph is not Ellipsis, \
'Assign your result to variable `mph`'
>>> assert speed_limit_mph is not Ellipsis, \
'Assign your result to variable `speed_limit_mph`'
>>> assert speed_limit_kph is not Ellipsis, \
'Assign your result to variable `speed_limit_kph`'
>>> assert type(kph) is float, \
'Variable `kph` has invalid type, should be float'
>>> assert type(mph) is float, \
'Variable `mph` has invalid type, should be float'
>>> assert type(speed_limit_mph) is float, \
'Variable `speed_limit_mph` has invalid type, should be float'
>>> assert type(speed_limit_kph) is float, \
'Variable `speed_limit_kph` has invalid type, should be float'
>>> round(kph, 3)
0.278
>>> round(mph, 3)
0.447
>>> round(speed_limit_mph, 1)
75.0
>>> round(speed_limit_kph, 1)
120.7
"""
SECOND = 1
MINUTE = 60 * SECOND
HOUR = 60 * MINUTE
m = 1
km = 1000 * m
mi = 1609.344 * m
# Miles per hour
# type: float
mph = ...
# Kilometers per hour
# type: float
kph = ...
# 75 miles per hour
# type: float
speed_limit = ...
# Speed limit in miles per hour
# type: float
speed_limit_mph = ...
# Speed limit in kilometers per hour
# type: float
speed_limit_kph = ...
3.2.9. Homework¶
"""
* Assignment: Type Float Pressure
* Type: homework
* Complexity: medium
* Lines of code: 2 lines
* Time: 3 min
English:
1. Operational pressure of EMU spacesuit: 4.3 PSI
2. Operational pressure of ORLAN spacesuit: 40 kPa
3. Calculate operational pressure in hPa for EMU
4. Calculate operational pressure in hPa for Orlan
5. Run doctests - all must succeed
Polish:
1. Ciśnienie operacyjne skafandra kosmicznego EMU (NASA): 4.3 PSI
2. Ciśnienie operacyjne skafandra kosmicznego ORLAN (Roscosmos): 40 kPa
3. Oblicz ciśnienie operacyjne skafandra EMU w hPa
4. Oblicz ciśnienie operacyjne skafandra Orlan w hPa
5. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert emu is not Ellipsis, \
'Assign your result to variable `emu`'
>>> assert orlan is not Ellipsis, \
'Assign your result to variable `orlan`'
>>> assert type(emu) is float, \
'Variable `emu` has invalid type, should be float'
>>> assert type(orlan) is float, \
'Variable `orlan` has invalid type, should be float'
>>> round(orlan, 1)
400.0
>>> round(emu, 1)
296.5
"""
Pa = 1
hPa = 100 * Pa
kPa = 1000 * Pa
psi = 6894.757 * Pa
# 4.3 pounds per square inch in hectopascals
# type: float
emu = ...
# 40 kilopascals in hectopascals
# type: float
orlan = ...
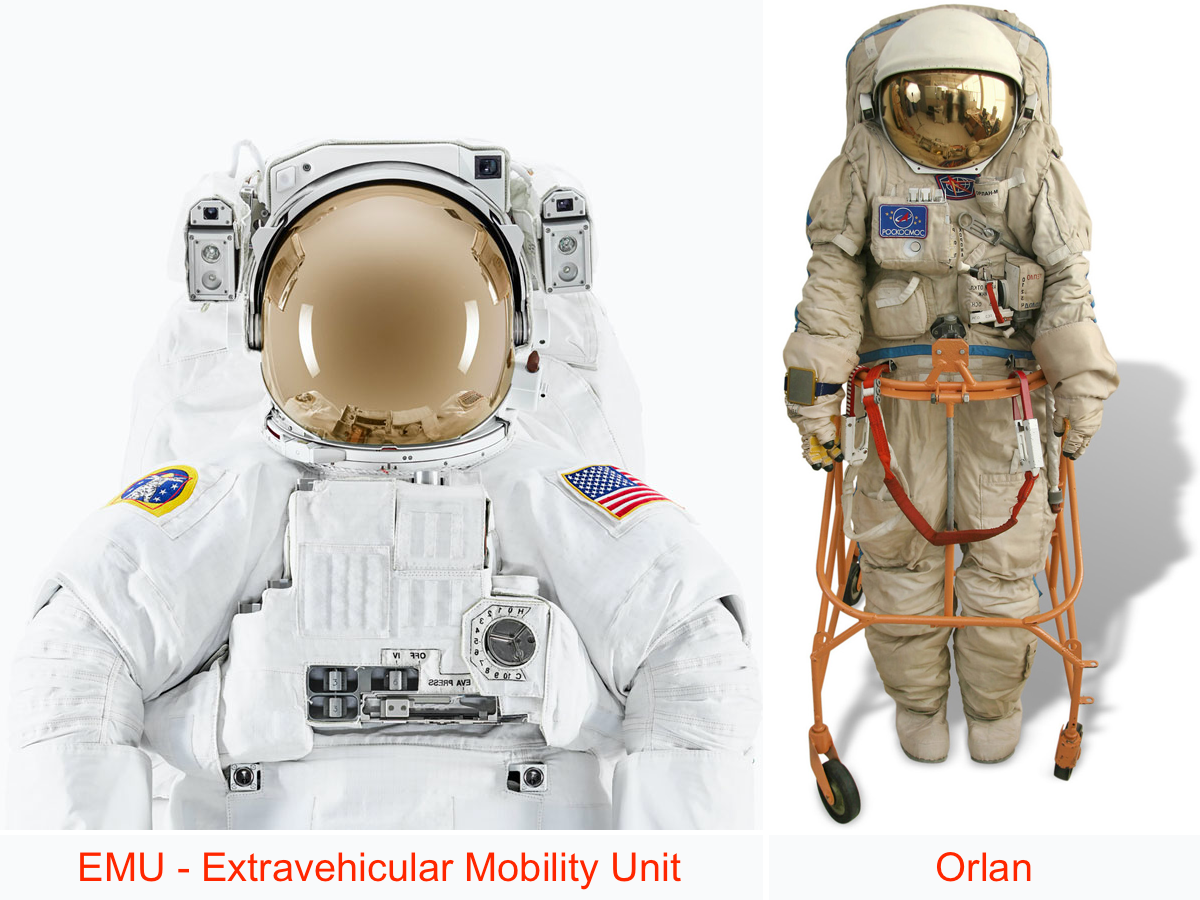
Figure 3.1. EMU and Orlan¶
"""
* Assignment: Type Float Percent
* Type: homework
* Complexity: medium
* Lines of code: 2 lines
* Time: 3 min
English:
1. International Standard Atmosphere (ISA) at sea level is
1 ata = 1013.25 hPa
2. Calculate `pO2` - partial pressure of Oxygen at sea level in hPa
3. To calculate partial pressure use ratio
(100% is 1013.25 hPa, 20.946% is how many hPa?)
4. Run doctests - all must succeed
Polish:
1. Międzynarodowa standardowa atmosfera (ISA) na poziomie morza wynosi
1 ata = 1013.25 hPa
2. Oblicz `pO2` - ciśnienie parcjalne tlenu na poziomie morza w hPa
3. Aby policzyć ciśnienie parcjalne skorzystaj z proporcji
(100% to 1013.25 hPa, 20.946% to ile hPa?)
4. Uruchom doctesty - wszystkie muszą się powieść
Hints:
* 1 hPa = 100 Pa
* 1 kPa = 1000 Pa
* 1 ata = 1013.25 hPa (ISA - International Standard Atmosphere)
* Atmosphere gas composition:
* Nitrogen 78.084%
* Oxygen 20.946%
* Argon 0.9340%
* Carbon Dioxide 0.0407%
* Others 0.001%
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert pO2 is not Ellipsis, \
'Assign your result to variable `pO2`'
>>> assert type(pO2) is float, \
'Variable `pO2` has invalid type, should be float'
>>> ata
101325.0
>>> round(pO2, 1)
212.2
"""
PERCENT = 100
N2 = 78.084 / PERCENT
O2 = 20.946 / PERCENT
Ar = 0.9340 / PERCENT
CO2 = 0.0407 / PERCENT
Others = 0.001 / PERCENT
Pa = 1
hPa = 100 * Pa
kPa = 1000 * Pa
# Standard atmosphere (ATA) is pressure at sea level: 1013.25 hectopascals
# type: float
ata = ...
# 20.946% of pressure at sea level in hPa
# type: float
pO2 = ...
"""
* Assignment: Type Float Gradient
* Type: homework
* Complexity: hard
* Lines of code: 7 lines
* Time: 8 min
English:
1. At what altitude above sea level, pressure is equal
to partial pressure of Oxygen
2. Print result in meters rounding to two decimal places
3. To calculate partial pressure use ratio
(100% is 1013.25 hPa, 20.946% is how many hPa?)
4. Calculated altitude is pressure at sea level minus
oxygen partial pressure divided by gradient
5. Mind the operator precedence
6. Run doctests - all must succeed
Polish:
1. Na jakiej wysokości nad poziomem morza panuje ciśnienie
równe ciśnieniu parcjalnemu tlenu?
2. Wypisz rezultat w metrach zaokrąglając do dwóch miejsc po przecinku
3. Aby policzyć ciśnienie parcjalne skorzystaj z proporcji
(100% to 1013.25 hPa, 20.946% to ile hPa?)
4. Wyliczona wysokość to ciśnienie atmosferyczne na poziomie morza minus
ciśnienie parcjalne tlenu podzielone przez gradient
5. Zwróć uwagę na kolejność wykonywania działań
6. Uruchom doctesty - wszystkie muszą się powieść
Hints:
* pressure gradient (decrease) = 11.3 Pa / 1 m
* 1 hPa = 100 Pa
* 1 kPa = 1000 Pa
* 1 ata = 1013.25 hPa (ISA - International Standard Atmosphere)
* Atmosphere gas composition:
* Nitrogen 78.084%
* Oxygen 20.946%
* Argon 0.9340%
* Carbon Dioxide 0.0407%
* Others 0.001%
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert pO2 is not Ellipsis, \
'Assign your result to variable `pO2`'
>>> assert gradient is not Ellipsis, \
'Assign your result to variable `gradient`'
>>> assert altitude is not Ellipsis, \
'Assign your result to variable `altitude`'
>>> assert type(pO2) is float, \
'Variable `pO2` has invalid type, should be float'
>>> assert type(gradient) is float, \
'Variable `gradient` has invalid type, should be float'
>>> assert type(altitude) is float, \
'Variable `altitude` has invalid type, should be float'
>>> pO2
21223.5345
>>> gradient
11.3
>>> round(altitude/m, 2)
7088.63
"""
PERCENT = 100
N2 = 78.084 / PERCENT
O2 = 20.946 / PERCENT
Ar = 0.9340 / PERCENT
CO2 = 0.0407 / PERCENT
Others = 0.001 / PERCENT
m = 1
Pa = 1
hPa = 100 * Pa
ata = 1013.25 * hPa
pO2 = O2 * ata
# 11.3 Pascals per meter
# type: float
gradient = ...
# Altitude is ata minus pO2 all that divided by gradient
# type: float
altitude = ...