6.4. Strategy¶
EN: Strategy
PL: Strategia
Type: object
6.4.1. Pattern¶
Similar to State Pattern
No single states
Can have multiple states
Different behaviors are represented by strategy objects
Store images with compressor and filters
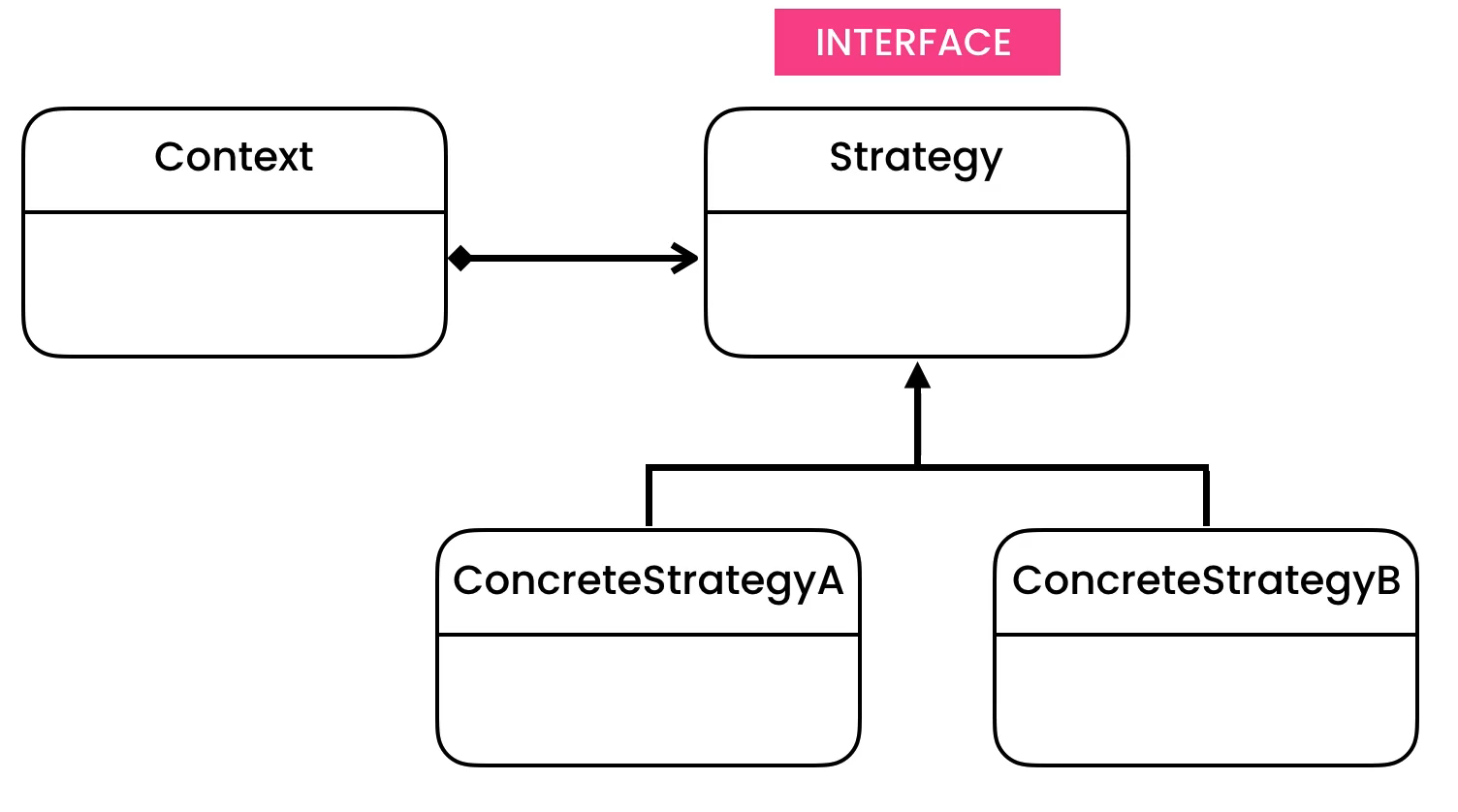
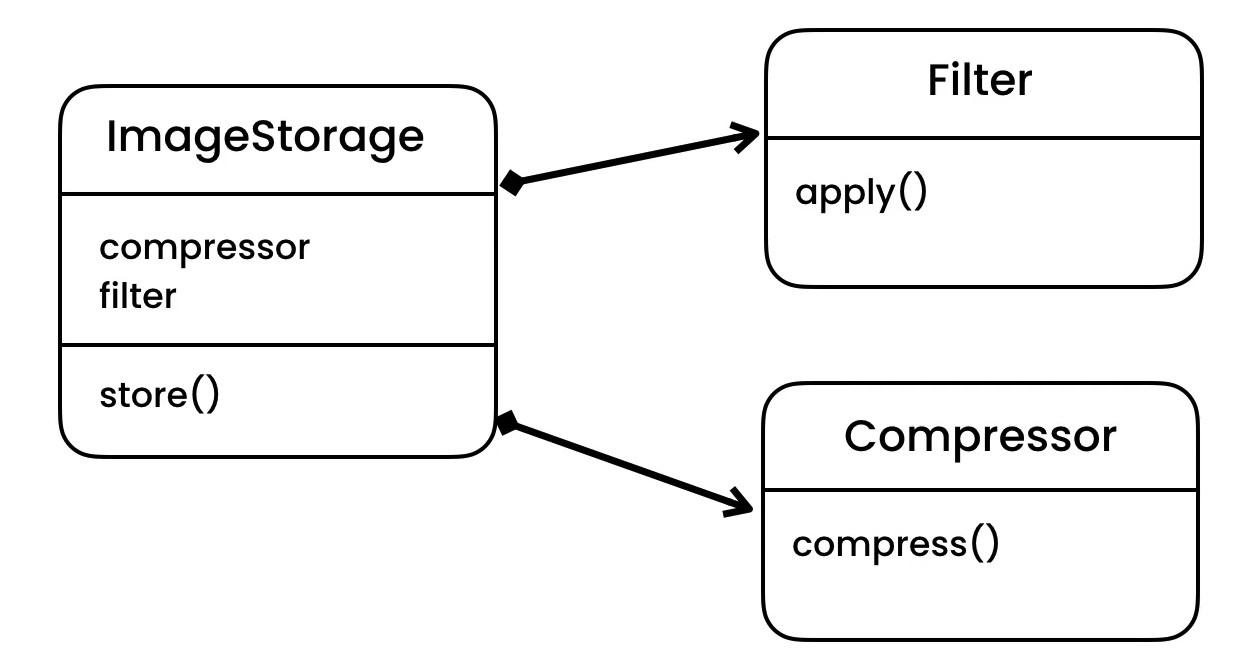
Figure 6.2. Strategy vs State Pattern¶
6.4.2. Problem¶
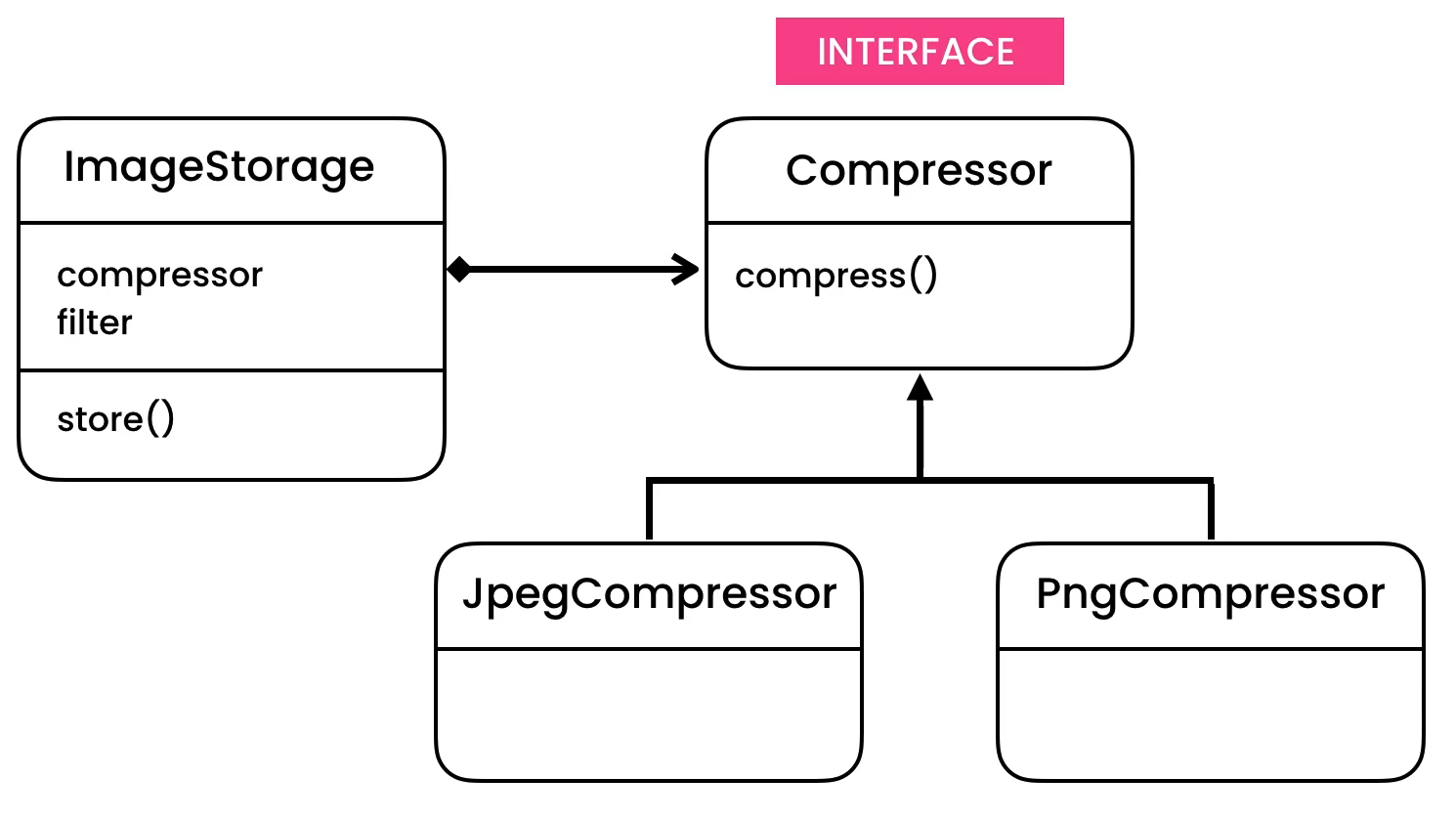
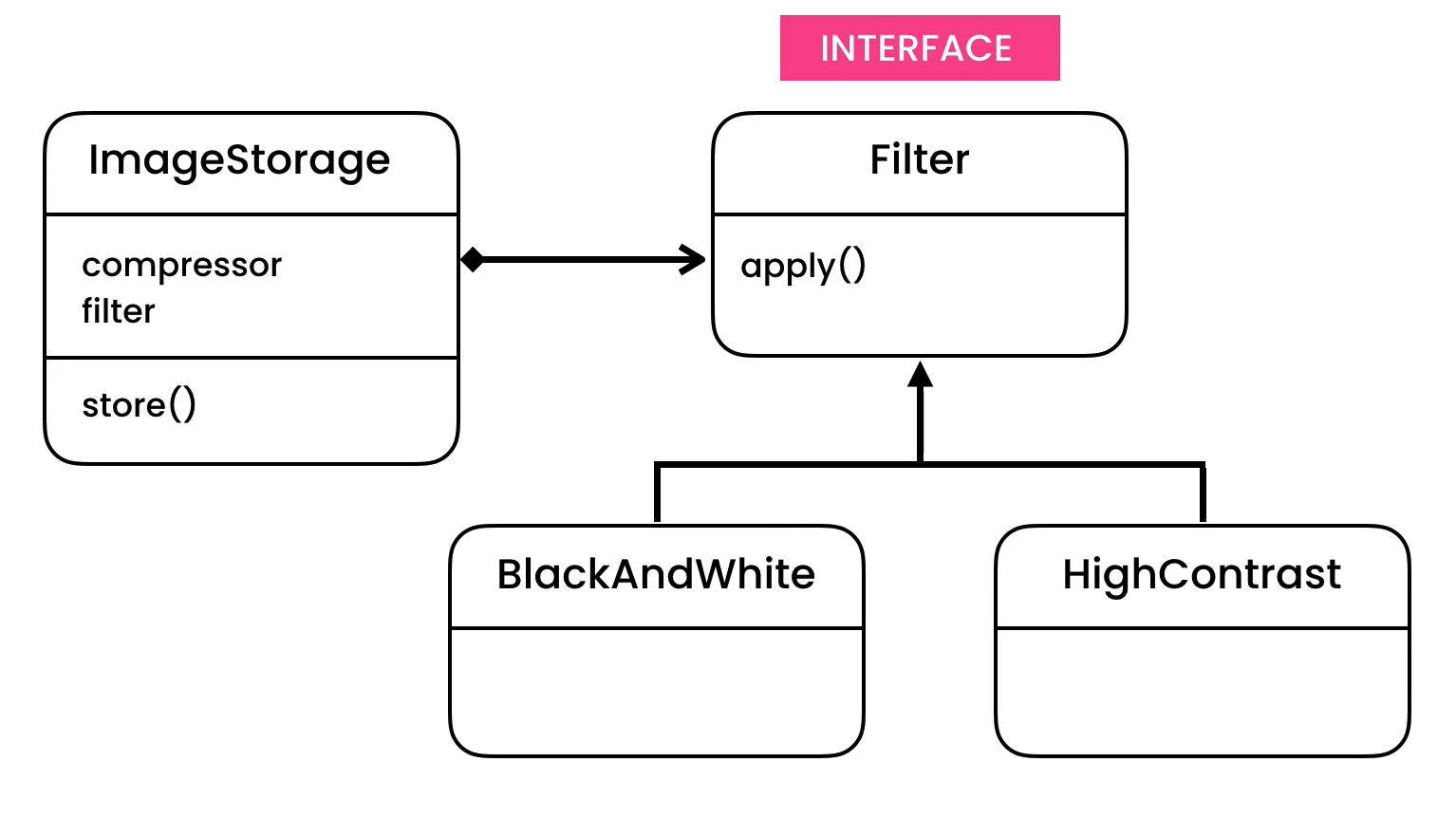
from dataclasses import dataclass
@dataclass
class ImageStorage:
compressor: str
filter: str
def store(self, filename) -> None:
if self.compressor == 'jpeg':
print('Compressing using JPEG')
elif self.compressor == 'png':
print('Compressing using PNG')
if self.filter == 'black&white':
print('Applying Black&White filter')
elif self.filter == 'high-contrast':
print('Applying high contrast filter')
6.4.3. Solution¶

from abc import ABC, abstractmethod
class Compressor(ABC):
@abstractmethod
def compress(self, filename: str) -> None:
pass
class JPEGCompressor(Compressor):
def compress(self, filename: str) -> None:
print('Compressing using JPEG')
class PNGCompressor(Compressor):
def compress(self, filename: str) -> None:
print('Compressing using PNG')
class Filter(ABC):
@abstractmethod
def apply(self, filename) -> None:
pass
class BlackAndWhiteFilter(Filter):
def apply(self, filename) -> None:
print('Applying Black and White filter')
class HighContrastFilter(Filter):
def apply(self, filename) -> None:
print('Applying high contrast filter')
class ImageStorage:
def store(self, filename: str, compressor: Compressor, filter: Filter) -> None:
compressor.compress(filename)
filter.apply(filename)
if __name__ == '__main__':
image_storage = ImageStorage()
image_storage.store('myfile.jpg', JPEGCompressor(), BlackAndWhiteFilter())
# Compressing using JPEG
# Applying Black and White filter
image_storage.store('myfile.png', PNGCompressor(), BlackAndWhiteFilter())
# Compressing using PNG
# Applying Black and White filter