3.2. Array Shape¶
Any shape operation changes only
np.ndarray.shape
andnp.ndarray.strides
and does not touch data
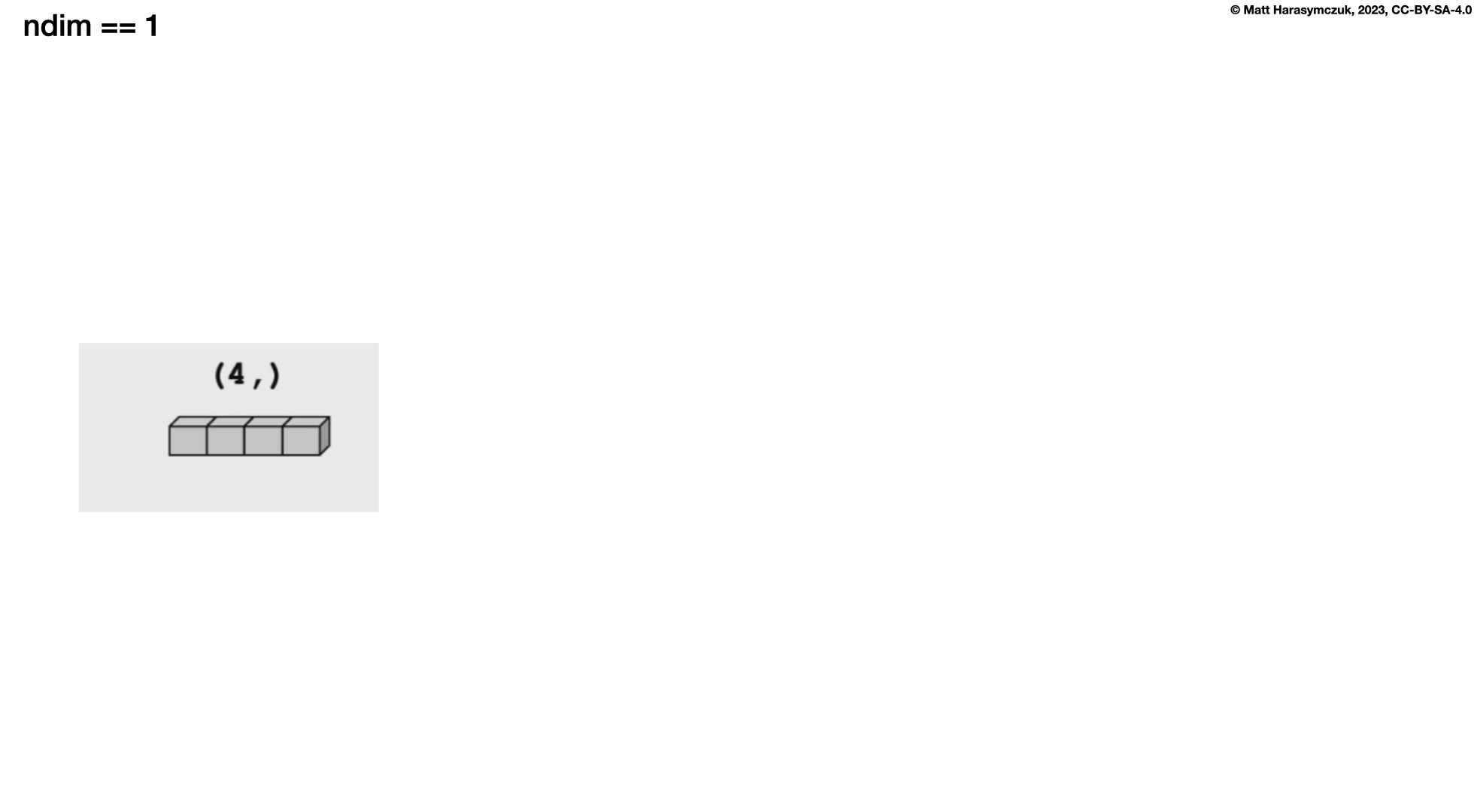
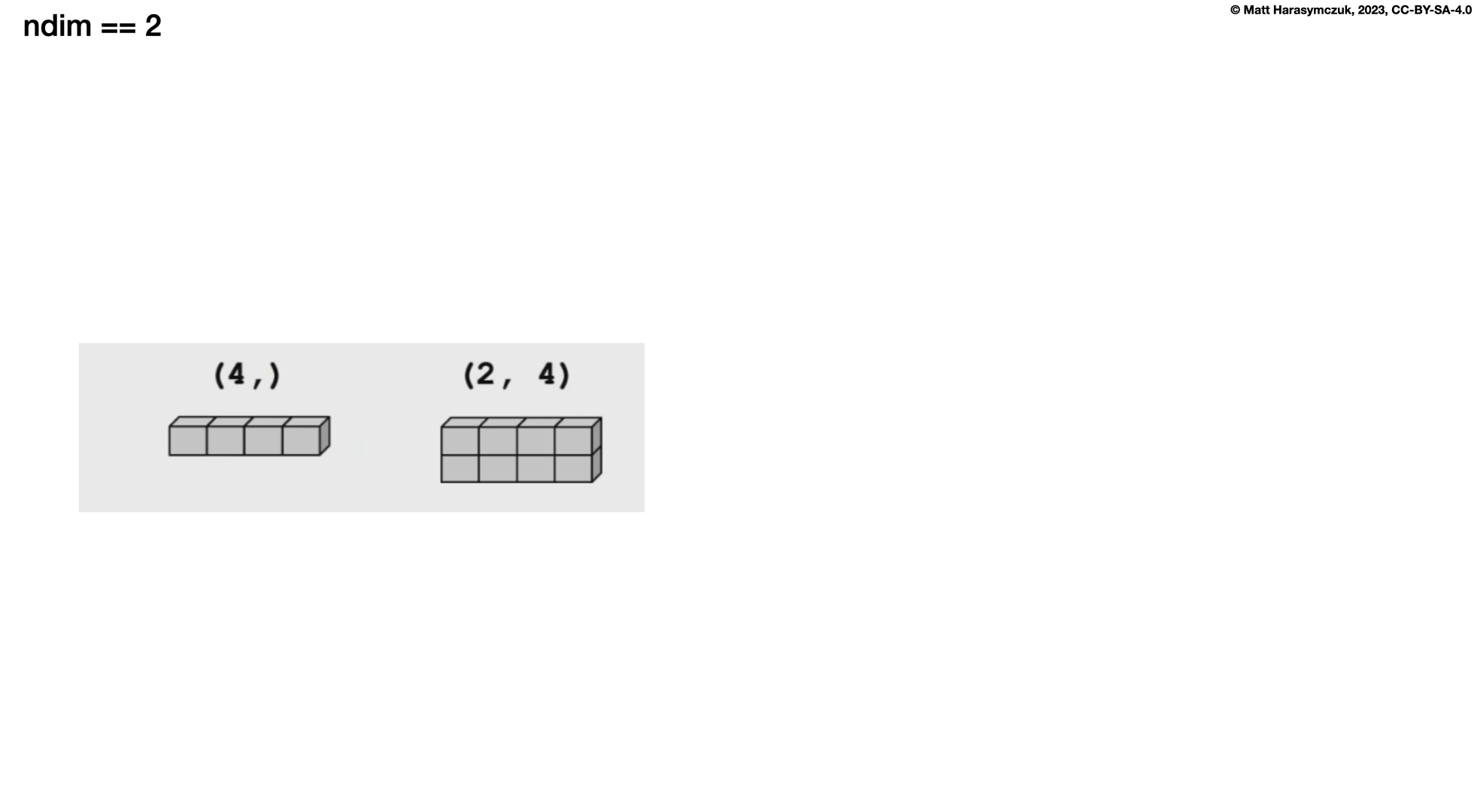
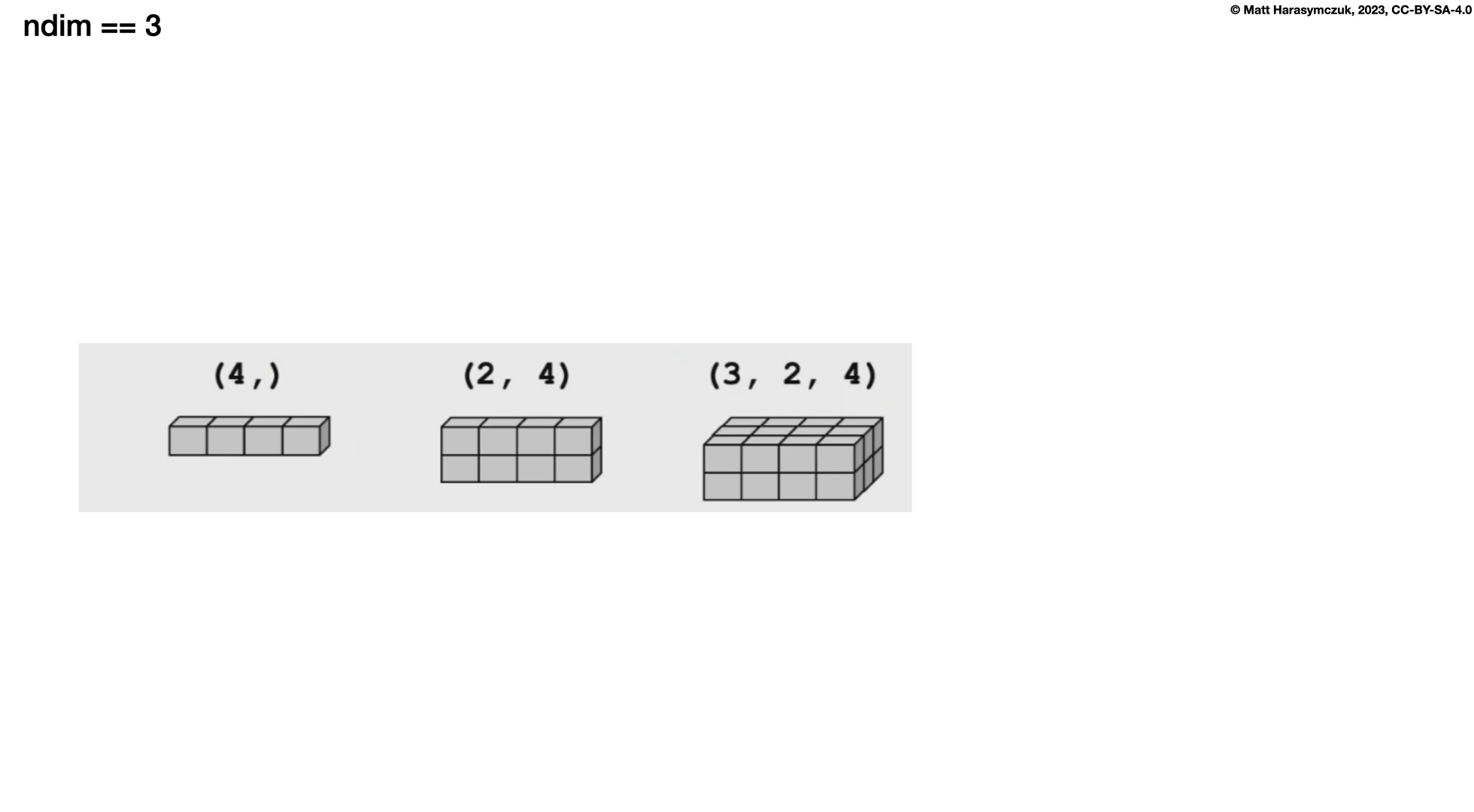
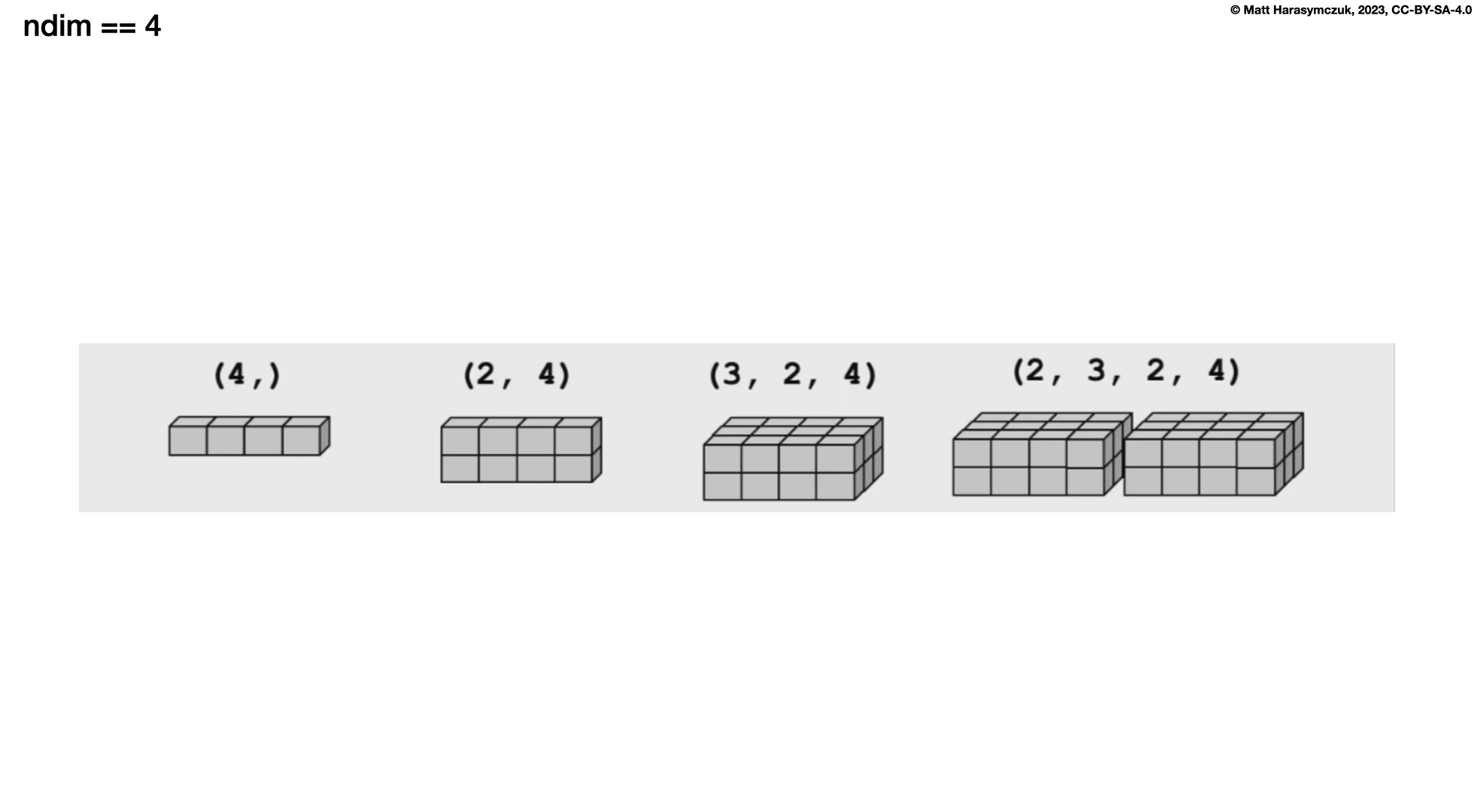
3.2.1. SetUp¶
>>> import numpy as np
3.2.2. Recap¶
>>> obj = [1, 2, 3]
>>>
>>> len(obj)
3
>>> obj1 = [1, 2, 3]
>>> obj2 = [4, 5, 6]
>>>
>>> len([obj1, obj2])
2
>>> len([ [1,2,3], [4,5,6] ])
2
>>> len([[1,2,3],
... [4,5,6]])
2
>>> obj1 = [1, 2, 3]
>>> obj2 = [4, 5, 6]
>>> obj3 = [7, 8, 9]
>>> obj4 = [10, 11, 12]
>>>
>>> len([ [obj1, obj2], [obj3, obj4] ])
2
>>> len([[obj1, obj2],
... [obj3, obj4]])
2
3.2.3. Shape¶
1-dimensional:
>>> a = np.array([1, 2, 3])
>>> a.shape
(3,)
2-dimensional:
>>> a = np.array([[1, 2, 3],
... [4, 5, 6]])
>>> a.shape
(2, 3)
>>> a = np.array([[1, 2, 3],
... [4, 5, 6],
... [7, 8, 9]])
>>> a.shape
(3, 3)
3-dimensional:
>>> a = np.array([[[ 1, 2, 3],
... [ 4, 5, 6],
... [ 5, 6, 7]],
... [[11, 22, 33],
... [44, 55, 66],
... [77, 88, 99]]])
>>> a.shape
(2, 3, 3)
3.2.4. Reshape¶
Returns new array
Does not modify inplace
a.reshape(1, 2)
is equivalent toa.reshape((1, 2))
>>> a = np.array([1, 2, 3])
>>>
>>> a.reshape(1, 3)
array([[1, 2, 3]])
>>>
>>> a.reshape(3, 1)
array([[1],
[2],
[3]])
>>> a = np.array([[1, 2, 3],
... [4, 5, 6]])
>>>
>>> a.reshape(3, 2)
array([[1, 2],
[3, 4],
[5, 6]])
>>>
>>> a.reshape(1, 6)
array([[1, 2, 3, 4, 5, 6]])
>>>
>>> a.reshape(6, 1)
array([[1],
[2],
[3],
[4],
[5],
[6]])
>>>
>>> a.reshape(5, 2)
Traceback (most recent call last):
ValueError: cannot reshape array of size 6 into shape (5,2)
>>> a = np.array([1, 2, 3, 4, 5, 6, 7, 8])
>>>
>>> a.reshape(2, 4)
array([[1, 2, 3, 4],
[5, 6, 7, 8]])
>>>
>>> a.reshape(2, 4, 1)
array([[[1],
[2],
[3],
[4]],
[[5],
[6],
[7],
[8]]])
>>>
>>> a.reshape(2, 2, 2)
array([[[1, 2],
[3, 4]],
[[5, 6],
[7, 8]]])
>>>
>>> a.reshape(1, 2, 4)
array([[[1, 2, 3, 4],
[5, 6, 7, 8]]])
>>>
>>> a.reshape(4, 2, 1)
array([[[1],
[2]],
[[3],
[4]],
[[5],
[6]],
[[7],
[8]]])
>>>
>>> a.reshape(1, 8, 1)
array([[[1],
[2],
[3],
[4],
[5],
[6],
[7],
[8]]])
>>>
>>> a.reshape(2, 3, 1)
Traceback (most recent call last):
ValueError: cannot reshape array of size 8 into shape (2,3,1)
3.2.5. Flatten¶
Returns new array (makes memory copy - expensive)
Does not modify inplace
>>> a = np.array([1, 2, 3])
>>>
>>> a.flatten()
array([1, 2, 3])
>>> a = np.array([[1, 2, 3],
... [4, 5, 6],
... [7, 8, 9]])
>>>
>>> a.flatten()
array([1, 2, 3, 4, 5, 6, 7, 8, 9])
>>> a = np.array([[[ 1, 2, 3],
... [ 4, 5, 6],
... [ 5, 6, 7]],
...
... [[11, 22, 33],
... [44, 55, 66],
... [77, 88, 99]]])
>>>
>>> a.flatten()
array([ 1, 2, 3, 4, 5, 6, 5, 6, 7, 11, 22, 33, 44, 55, 66, 77, 88, 99])
3.2.6. Ravel¶
Ravel is the same as Flatten but returns a reference (or view) of the array if possible (i.e. memory is contiguous)
Otherwise returns new array (makes memory copy)
>>> a = np.array([1, 2, 3])
>>>
>>> a.ravel()
array([1, 2, 3])
>>> a = np.array([[1, 2, 3],
... [4, 5, 6],
... [7, 8, 9]])
>>>
>>> a.ravel()
array([1, 2, 3, 4, 5, 6, 7, 8, 9])
>>> a = np.array([[[ 1, 2, 3],
... [ 4, 5, 6],
... [ 5, 6, 7]],
...
... [[11, 22, 33],
... [44, 55, 66],
... [77, 88, 99]]])
>>>
>>>
>>> a.ravel()
array([ 1, 2, 3, 4, 5, 6, 5, 6, 7, 11, 22, 33, 44, 55, 66, 77, 88, 99])
3.2.7. Flatten vs Ravel¶
>>> a = np.array([1, 2, 3])
>>> b = a.ravel()
>>> c = a.flatten()
>>> a[0] = 99
>>> a # original
array([99, 2, 3])
>>>
>>> b # flatten
array([99, 2, 3])
>>>
>>> c # ravel
array([1, 2, 3])
3.2.8. Recap¶
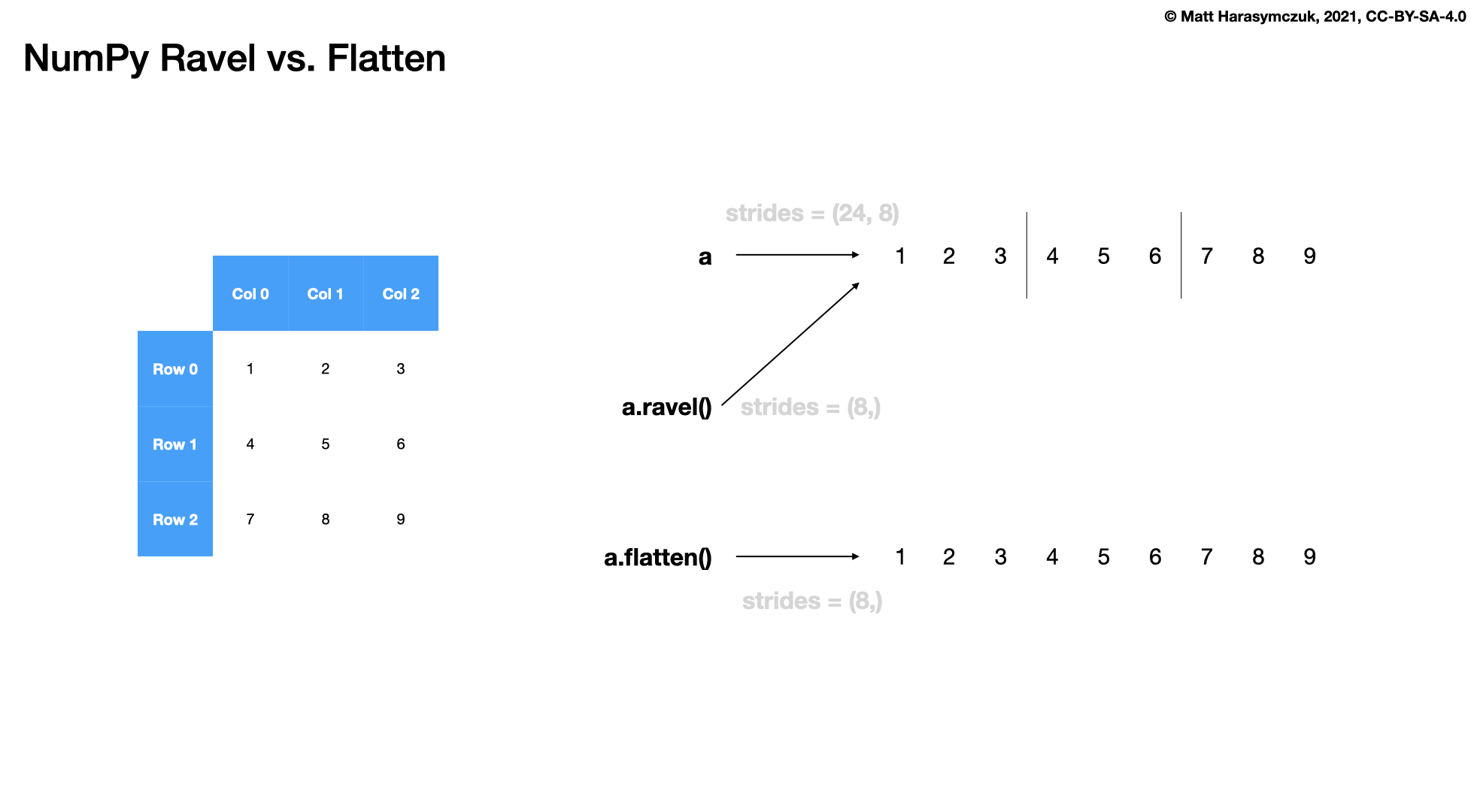
3.2.9. Assignments¶
"""
* Assignment: Numpy Shape 1d
* Complexity: easy
* Lines of code: 2 lines
* Time: 3 min
English:
1. Define `result_ravel` with result of flattening `DATA` using `.ravel()` method
2. Define `result_flatten` with result of flattening `DATA` using `.flatten()` method
3. Define `result_reshape` with result of reshaping `DATA` into 1x9
4. Run doctests - all must succeed
Polish:
1. Zdefiniuj `result_ravel` z wynikiem spłaszczenia `DATA` używając metody `.ravel()`
2. Zdefiniuj `result_flatten` z wynikiem spłaszczenia `DATA` używając metody `.flatten()`
3. Zdefiniuj `result_reshape` z wynikiem zmiany kształtu `DATA` na 1x9
4. Uruchom doctesty - wszystkie muszą się powieść
Tests:
>>> import sys; sys.tracebacklimit = 0
>>> assert result_ravel is not Ellipsis, \
'Assign result to variable: `result_ravel`'
>>> assert type(result_ravel) is np.ndarray, \
'Variable `result_ravel` has invalid type, expected: np.ndarray'
>>> assert result_flatten is not Ellipsis, \
'Assign result to variable: `result_flatten`'
>>> assert type(result_flatten) is np.ndarray, \
'Variable `result_flatten` has invalid type, expected: np.ndarray'
>>> assert result_reshape is not Ellipsis, \
'Assign result to variable: `result_reshape`'
>>> assert type(result_reshape) is np.ndarray, \
'Variable `result_reshape` has invalid type, expected: np.ndarray'
>>> result_flatten
array([1, 2, 3, 4, 5, 6, 7, 8, 9])
>>> result_ravel
array([1, 2, 3, 4, 5, 6, 7, 8, 9])
>>> result_reshape
array([[1, 2, 3, 4, 5, 6, 7, 8, 9]])
"""
import numpy as np
DATA = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
result_ravel = ...
result_flatten = ...
result_reshape = ...